资源简介
用C语言实现的Butterworth滤波器,附带滤波数据,VC6.0控制台程序。
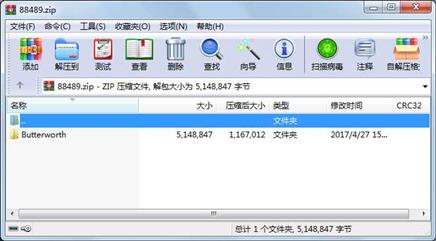
代码片段和文件信息
#include
#include
#include
#include
#include
#include
using namespace std;
#define pi ((double)3.1415926)
struct DESIGN_SPECIFICATION
{
double Passband;
double Stopband;
double Passband_attenuation;
double Stopband_attenuation;
};
//复数乘
_complex ComplexMul( _complex a _complex b )
{
_complex c;
c.x = a.x*b.x - a.y*b.y;
c.y = a.y*b.x + a.x*b.y;
return c;
}
//复数除
_complex ComplexDiv( _complex a _complex b)
{
_complex c;
double dTmp = b.x * b.x + b.y * b.y;
//除数太小防溢出
if( dTmp < 1.0E-300 ){
c.x = 1.0E300;
c.y = 1.0E300;
return c;
}
c.x = ( a.x * b.x + a.y * b.y) / dTmp;
c.y = ( a.x * b.y - a.y * b.x) / dTmp;
return c;
}
//计算滤波器阶数N
int Buttord(double Passband
double Stopband
double Passband_attenuation
double Stopband_attenuation)
{
int N;
printf(“Wp = %lf [rad/sec] \n“ Passband);
printf(“Ws = %lf [rad/sec] \n“ Stopband);
printf(“Ap = %lf [dB] \n“ Passband_attenuation);
printf(“As = %lf [dB] \n“ Stopband_attenuation);
printf(“--------------------------------------------------------\n“ );
N = ceil(0.5*( log10 (( pow (10 Stopband_attenuation/10) - 1)/
( pow (10 Passband_attenuation/10) - 1)) / log10 (Stopband/Passband) ));
return (int)N;
}
//计算S平面的滤波器系数
int Butter(int N double Cutoff double *a double *b)
{
double dk = 0;
int k = 0;
int count = 0count_1 = 0;
_complex *poles *Res *Res_Save;
poles = new _complex[N]();
Res = new _complex[N+1]();
Res_Save = new _complex[N+1]();
memset(poles 0 sizeof(_complex)*(N));
memset(Res 0 sizeof(_complex)*(N+1));
memset(Res_Save 0 sizeof(_complex)*(N+1));
if((N % 2) == 0) dk = 0.5;
else dk = 0;
for(k = 0;k <= ((2*N)-1) ; k++)
{
if(Cutoff*cos((2*k+N-1)*(pi/(2*N))) < 0.0)
{
poles[count].x = -Cutoff*cos((2*k+N-1)*(pi/(2*N)));
poles[count].y = -Cutoff*sin((2*k+N-1)*(pi/(2*N)));
count++;
if (count == N) break;
}
}
printf(“Pk = \n“ );
for(count = 0; count < N; count++)
{
printf(“(%lf) + (%lf i) \n“ -poles[count].x -poles[count].y);
}
printf(“--------------------------------------------------------\n“ );
Res[0].x = poles[0].x;
Res[0].y = poles[0].y;
Res[1].x = 1;
Res[1].y= 0;
for(count_1 = 0;count_1 < N-1;count_1++)//N个极点相乘次数
{
for(count = 0;count <= count_1 + 2;count++)
{
if(0 == count)
{
Res_Save[count] = ComplexMul( Res[count] poles[count_1+1] );
}
else if((count_1 + 2) == count)
{
Res_Save[count].x += Res[count - 1].x;
Res_Save[count].y += Res[count - 1].y;
}
else
{
Res_Save[count] = Comple
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2017-04-27 15:55 Butterworth\
文件 48322 2017-04-06 09:27 Butterworth\1.txt
文件 145792 2017-03-27 19:25 Butterworth\123.txt
文件 149793 2017-04-01 15:24 Butterworth\1234.txt
文件 106912 2017-05-01 10:16 Butterworth\butter1.txt
文件 4335 2017-04-06 21:29 Butterworth\Butterworth.dsp
文件 530 2017-04-04 19:34 Butterworth\Butterworth.dsw
文件 41984 2017-05-01 10:16 Butterworth\Butterworth.ncb
文件 53760 2017-05-01 10:16 Butterworth\Butterworth.opt
文件 1165 2017-05-01 10:16 Butterworth\Butterworth.plg
目录 0 2017-04-27 15:55 Butterworth\Debug\
文件 254976 2017-05-01 10:16 Butterworth\Debug\Butterworth.bsc
文件 315455 2017-05-01 10:16 Butterworth\Debug\Butterworth.exe
文件 418012 2017-05-01 10:16 Butterworth\Debug\Butterworth.ilk
文件 2095684 2017-05-01 10:10 Butterworth\Debug\Butterworth.pch
文件 1131520 2017-05-01 10:16 Butterworth\Debug\Butterworth.pdb
文件 28267 2017-05-01 10:16 Butterworth\Debug\main.obj
文件 0 2017-05-01 10:16 Butterworth\Debug\main.sbr
文件 123904 2017-05-01 10:16 Butterworth\Debug\vc60.idb
文件 110592 2017-05-01 10:16 Butterworth\Debug\vc60.pdb
文件 10932 2017-05-01 10:16 Butterworth\main.cpp
文件 106912 2017-04-06 09:28 Butterworth\output22.txt
- 上一篇:基于QT的简单文本编辑器
- 下一篇:人工智能归结反演c++代码
相关资源
- 中值滤波C语言154954
- 经典滤波算法
- 图像预处理五种滤波
- 粒子滤波器+目标跟踪的C++实现,VS2
- 中值滤波_均值滤波c语言实现_工程文
- 基于MFC的界面设计,包括灰度化、边
- 数字图像处理实验源代码中值滤波,
- RGB图像中值滤波源码
- 各种Kalman滤波的比较程序——C++/MFC版
- opencv卡尔曼滤波
- filter_solutions_设计滤波器笔记总结
- 数字信号处理c语言程序集-各种数字信
- 无源滤波器计算
- rar文件MFC 图像处理之图像增强 图像平
- Nuhertz Filter Solutions破解版
- 巴特沃斯高通滤波器
- OpenCV图像模糊程序 by浅墨
- 数字图像处理中值,均值滤波
- 滤波程序滤波程序滤波程序c++语言编
- VC实现图像高斯/中值/均值/双边/滤波
- 傅里叶变换研究|C++源码|带通滤波器
- FIr滤波器的设计基于VC++
- 基于网格的坡度滤波C++程序
- 非线性滤波
- MFC图像直方图均衡化、锐化、中值滤
- 单片机50Hz数字滤波器
- 扩展卡尔曼滤波项目C++代码
- 双边滤波器C++ 代码
- 粒子滤波跟踪程序源代码
- 图像滤波常用算法
评论
共有 条评论