资源简介
src文件夹下存放SOIL.h头文件,X64文件夹下分别存放debug和release版本下的lib文件,自行提取
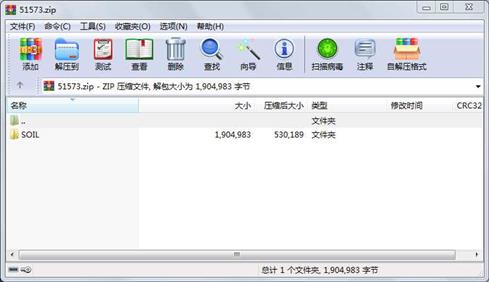
代码片段和文件信息
/*
Jonathan Dummer
2007-07-31-10.32
simple DXT compression / decompression code
public domain
*/
#include “image_DXT.h“
#include
#include
#include
#include
/* set this =1 if you want to use the covarince matrix method...
which is better than my method of using standard deviations
overall except on the infintesimal chance that the power
method fails for finding the largest eigenvector */
#define USE_COV_MAT 1
/********* Function Prototypes *********/
/*
Takes a 4x4 block of pixels and compresses it into 8 bytes
in DXT1 format (color only no alpha). Speed is valued
over prettyness at least for now.
*/
void compress_DDS_color_block(
int channels
const unsigned char *const uncompressed
unsigned char compressed[8] );
/*
Takes a 4x4 block of pixels and compresses the alpha
component it into 8 bytes for use in DXT5 DDS files.
Speed is valued over prettyness at least for now.
*/
void compress_DDS_alpha_block(
const unsigned char *const uncompressed
unsigned char compressed[8] );
/********* Actual Exposed Functions *********/
int
save_image_as_DDS
(
const char *filename
int width int height int channels
const unsigned char *const data
)
{
/* variables */
FILE *fout;
unsigned char *DDS_data;
DDS_header header;
int DDS_size;
/* error check */
if( (NULL == filename) ||
(width < 1) || (height < 1) ||
(channels < 1) || (channels > 4) ||
(data == NULL ) )
{
return 0;
}
/* Convert the image */
if( (channels & 1) == 1 )
{
/* no alpha just use DXT1 */
DDS_data = convert_image_to_DXT1( data width height channels &DDS_size );
} else
{
/* has alpha so use DXT5 */
DDS_data = convert_image_to_DXT5( data width height channels &DDS_size );
}
/* save it */
memset( &header 0 sizeof( DDS_header ) );
header.dwMagic = (‘D‘ << 0) | (‘D‘ << 8) | (‘S‘ << 16) | (‘ ‘ << 24);
header.dwSize = 124;
header.dwFlags = DDSD_CAPS | DDSD_HEIGHT | DDSD_WIDTH | DDSD_PIXELFORMAT | DDSD_LINEARSIZE;
header.dwWidth = width;
header.dwHeight = height;
header.dwPitchOrLinearSize = DDS_size;
header.sPixelFormat.dwSize = 32;
header.sPixelFormat.dwFlags = DDPF_FOURCC;
if( (channels & 1) == 1 )
{
header.sPixelFormat.dwFourCC = (‘D‘ << 0) | (‘X‘ << 8) | (‘T‘ << 16) | (‘1‘ << 24);
} else
{
header.sPixelFormat.dwFourCC = (‘D‘ << 0) | (‘X‘ << 8) | (‘T‘ << 16) | (‘5‘ << 24);
}
header.sCaps.dwCaps1 = DDSCAPS_TEXTURE;
/* write it out */
fout = fopen( filename “wb“);
fwrite( &header sizeof( DDS_header ) 1 fout );
fwrite( DDS_data 1 DDS_size fout );
fclose( fout );
/* done */
free( DDS_data );
return 1;
}
unsigned char* convert_image_to_DXT1(
const unsigned char *const uncompressed
int width int height int channels
int *out_size )
{
unsigned char *compressed;
int i j x y;
unsigned char ublock[16*3];
unsigned cha
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2017-12-17 00:10 SOIL\src\
文件 17181 2017-02-21 12:47 SOIL\src\image_DXT.c
文件 3212 2017-02-21 12:47 SOIL\src\image_DXT.h
文件 10511 2017-02-21 12:47 SOIL\src\image_helper.c
文件 2287 2017-02-21 12:47 SOIL\src\image_helper.h
目录 0 2017-12-17 00:10 SOIL\src\original\
文件 118175 2017-02-21 12:47 SOIL\src\original\stb_image-1.09.c
文件 126186 2017-02-21 12:47 SOIL\src\original\stb_image-1.16.c
文件 58744 2017-02-21 12:47 SOIL\src\SOIL.c
文件 15545 2017-02-21 12:47 SOIL\src\SOIL.h
文件 117420 2017-02-21 12:47 SOIL\src\stb_image_aug.c
文件 16945 2017-02-21 12:47 SOIL\src\stb_image_aug.h
文件 797 2017-02-21 12:47 SOIL\src\stbi_DDS_aug.h
文件 15447 2017-02-21 12:47 SOIL\src\stbi_DDS_aug_c.h
文件 11270 2017-02-21 12:47 SOIL\src\test_SOIL.cpp
目录 0 2017-12-17 00:10 SOIL\Win32\
目录 0 2017-12-17 00:10 SOIL\Win32\Debug\
目录 0 2017-12-17 00:10 SOIL\Win32\Debug\ALL_BUILD\
文件 556 2017-02-21 12:47 SOIL\Win32\Debug\ALL_BUILD\ALL_BUILD.log
目录 0 2017-12-17 00:10 SOIL\Win32\Debug\ALL_BUILD\ALL_BUILD.tlog\
文件 175 2017-02-21 12:47 SOIL\Win32\Debug\ALL_BUILD\ALL_BUILD.tlog\ALL_BUILD.lastbuildstate
文件 992 2017-02-21 12:47 SOIL\Win32\Debug\ALL_BUILD\ALL_BUILD.tlog\custombuild.command.1.tlog
文件 4390 2017-02-21 12:47 SOIL\Win32\Debug\ALL_BUILD\ALL_BUILD.tlog\custombuild.read.1.tlog
文件 278 2017-02-21 12:47 SOIL\Win32\Debug\ALL_BUILD\ALL_BUILD.tlog\custombuild.write.1.tlog
目录 0 2017-12-17 00:10 SOIL\Win32\Debug\ZERO_CHECK\
文件 492 2017-02-21 12:47 SOIL\Win32\Debug\ZERO_CHECK\ZERO_CHECK.log
目录 0 2017-12-17 00:10 SOIL\Win32\Debug\ZERO_CHECK\ZERO_CHECK.tlog\
文件 1136 2017-02-21 12:47 SOIL\Win32\Debug\ZERO_CHECK\ZERO_CHECK.tlog\custombuild.command.1.tlog
文件 4604 2017-02-21 12:47 SOIL\Win32\Debug\ZERO_CHECK\ZERO_CHECK.tlog\custombuild.read.1.tlog
文件 356 2017-02-21 12:47 SOIL\Win32\Debug\ZERO_CHECK\ZERO_CHECK.tlog\custombuild.write.1.tlog
文件 175 2017-02-21 12:47 SOIL\Win32\Debug\ZERO_CHECK\ZERO_CHECK.tlog\ZERO_CHECK.lastbuildstate
............此处省略81个文件信息
- 上一篇:铭瑄580 8G 镁光颗粒
- 下一篇:自定义端口扫描工具
相关资源
- OpenGL参考手册
- 联想y470无线网卡驱动 for 32位64位
- JDK8 绿色版 免安装版 64位
- CAD2010注册机32bit and 64bit
- JM阅读笔记(学习H264)
- Qt Creator opengl实现四元数鼠标控制轨迹
- 12864滚动显示汉字
- OpenGL文档,api大全,可直接查询函数
- opengl轮廓字体源代码
- MFC读三维模型obj文件
- 利用OpenGL写毛笔字算法
- MFC中OpenGL面和体的绘制以及动画效果
- mexLasso.m 及mexLasso.mexw64
- keil vcom windows 7 64bit 驱动
- 基于OPENGL的光线跟踪源代码368758
- h264 ip核,经过asic验证
- VC 实现三维旋转(源码)
- 单片机控制74ls164程序
- lcd12864的VHDL程序
- 自编用openGL实现3D分形树,分形山
- OpenGL球形贴图自旋程序
- 全自动多功能编码转换工具(URLASCI
- LCD12864滚动显示
- zlib 最新 1.2.8 win32 win64 编译好的dll
- OpenGL导入贴图的Texture类
- atmega64bootload
- 计算机图形学(openGL)代码
- H.264编码器流程图
- 用OpenGL开发的机械臂运动仿真程序(
- OpenGL-3D坦克模拟
评论
共有 条评论