资源简介
实现乐鑫esp8266的无线OTA升级,实现远程在线升级固件。
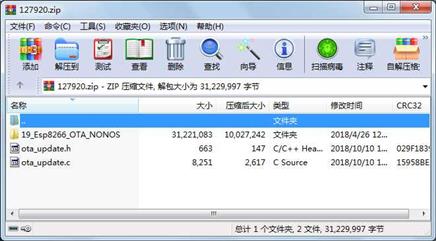
代码片段和文件信息
/******************************************************************************/
/* ESP8266 Family */
/* JiaXin */
/******************************************************************************/
#include “osapi.h“
#include “mem.h“
#include “upgrade.h“
#include “user_interface.h“
#include “ota_update.h“
#include “espconn.h“
struct EspconnUpdateTransferData {
//// 请求参数 ////
unsigned short remotePort;
char* host;
char* filename;
};
#define INET_ADDRSTRLEN 18
////////////////////////////////////////////////////////////////////////////////
bool ICACHE_FLASH_ATTR getIpArray(char* host char *server_ip)
{
if(NULL == server_ip) {
return false;
}
char tmp[4];
char *ptr = NULL;
char *divStr = “.“;
int i = 0;
ptr = strtok(host divStr);
while (ptr != NULL) {
strncpy(tmp ptr strlen(ptr));
int ip_int = atoi(tmp);
if (ip_int < 0 || ip_int > 255) {
return false;
}
server_ip[i] = ip_int;
ptr = strtok(NULL divStr);
if(NULL != ptr) {
++i;
}
}
if(i != 3) {
return false;
}
char output[32];
os_sprintf(output “getIpAddr(): “IPSTR“\r\n“ IP2STR(server_ip));
uart0_sendStr(output);
return true;
}
void ICACHE_FLASH_ATTR getIpAddrString(ip_addr_t *ipaddr char *ip)
{
memset(ip 0 INET_ADDRSTRLEN);
os_sprintf(ip IPSTR ip4_addr1(ipaddr) ip4_addr2(ipaddr) ip4_addr3(ipaddr) ip4_addr4(ipaddr));
}
bool ICACHE_FLASH_ATTR http_parse_request_url(char *URL char *host int hostSizechar *filename int nameSize unsigned short *port) {
char *PA;
char *PB;
memset(host 0 hostSize);
memset(filename 0 nameSize);
*port = 0;
if (!(*URL)){
uart0_sendStr(“\r\n ----- URL return ----- \r\n“);
return false;
}
PA = URL;
if (!strncmp(PA “http://“ strlen(“http://“))) {
PA = URL + strlen(“http://“);
} else if (!strncmp(PA “https://“ strlen(“https://“))) {
PA = URL + strlen(“https://“);
}
PB = strchr(PA ‘/‘);
if (PB) {
uart0_sendStr(“\r\n ----- PB=true ----- \r\n“);
memcpy(host PA strlen(PA) - strlen(PB));
if (PB + 1) {
memcpy(filename PB + 1 strlen(PB - 1));
filename[strlen(PB) - 1] = 0;
}
host[strlen(PA) - strlen(PB)] = 0;
//uart0_log(host);
} else {
uart0_sendStr(“\r\n ----- PB=false ----- \r\n“);
memcpy(host PA strlen(PA));
host[strlen(PA)] = 0;
//uart0_log(host);
}
PA = strchr(host ‘:‘);
if (PA){
*port = atoi(PA + 1);
host[PA - host] = 0;
}else{
*port = 80;
}
char buf[500];
os_sprintf(buf “---PARSE URL host[%s] filename[%s] port[%u]\r\n“ host filename *port);
uart0_sendStr(buf);
return true;
}
////////////////////////////////////////////////////////////////////////////////
void ICACHE_FLASH_ATTR freeTcpConn(struct espconn *pTcp_conn)
{
if(NULL != pTcp_conn) {
struct EspconnUpdateTransferData * pData = pTcp_conn->reverse;
if(NULL != pData) {
if(NULL != pData->filename) {
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 8251 2018-10-10 11:25 ota_update.c
文件 663 2018-10-10 10:41 ota_update.h
目录 0 2018-04-26 12:06 19_Esp8266_OTA_NONOS\
文件 3719 2018-04-26 12:06 19_Esp8266_OTA_NONOS\.cproject
文件 798 2018-04-26 12:06 19_Esp8266_OTA_NONOS\.project
目录 0 2018-04-26 12:06 19_Esp8266_OTA_NONOS\.settings\
目录 0 2018-04-27 11:38 19_Esp8266_OTA_NONOS\app\
目录 0 2018-04-26 12:06 19_Esp8266_OTA_NONOS\app\.output\
目录 0 2018-04-27 11:38 19_Esp8266_OTA_NONOS\app\.output\eagle\
目录 0 2018-04-27 11:38 19_Esp8266_OTA_NONOS\app\.output\eagle\debug\
目录 0 2018-04-27 11:38 19_Esp8266_OTA_NONOS\app\.output\eagle\debug\bin\
目录 0 2018-04-27 11:38 19_Esp8266_OTA_NONOS\app\.output\eagle\debug\image\
文件 337118 2018-04-27 11:38 19_Esp8266_OTA_NONOS\app\.output\eagle\debug\image\eagle.app.v6.out
文件 3080 2018-02-06 04:45 19_Esp8266_OTA_NONOS\app\gen_misc.bat
文件 3788 2018-02-06 04:45 19_Esp8266_OTA_NONOS\app\gen_misc.sh
目录 0 2018-04-26 12:04 19_Esp8266_OTA_NONOS\app\include\
文件 1296 2018-02-06 04:45 19_Esp8266_OTA_NONOS\app\include\user_config.h
文件 2814 2018-02-06 04:45 19_Esp8266_OTA_NONOS\app\Makefile
目录 0 2018-04-26 14:41 19_Esp8266_OTA_NONOS\app\user\
目录 0 2018-04-26 12:06 19_Esp8266_OTA_NONOS\app\user\.output\
目录 0 2018-04-27 11:38 19_Esp8266_OTA_NONOS\app\user\.output\eagle\
目录 0 2018-04-27 11:38 19_Esp8266_OTA_NONOS\app\user\.output\eagle\debug\
目录 0 2018-04-27 11:38 19_Esp8266_OTA_NONOS\app\user\.output\eagle\debug\lib\
文件 22956 2018-04-27 11:38 19_Esp8266_OTA_NONOS\app\user\.output\eagle\debug\lib\libuser.a
目录 0 2018-04-27 11:38 19_Esp8266_OTA_NONOS\app\user\.output\eagle\debug\obj\
文件 1317 2018-04-27 11:38 19_Esp8266_OTA_NONOS\app\user\.output\eagle\debug\obj\user_main.d
文件 22692 2018-04-27 11:38 19_Esp8266_OTA_NONOS\app\user\.output\eagle\debug\obj\user_main.o
文件 1477 2018-02-06 04:45 19_Esp8266_OTA_NONOS\app\user\Makefile
文件 7560 2018-04-27 11:37 19_Esp8266_OTA_NONOS\app\user\user_main.c
目录 0 2018-04-26 14:49 19_Esp8266_OTA_NONOS\bin\
目录 0 2018-02-06 04:45 19_Esp8266_OTA_NONOS\bin\at\
............此处省略114个文件信息
- 上一篇:PowerBI视觉对象75个
- 下一篇:佳蓝客户端在线生成平台
相关资源
- STC8951系列单片机中方指南
- 基于MCS_51单片机的工业屏柜散热方案
- MCS_51单片机与8255A的接口设计
- 飞思卡尔单片机MC9S12XS12G128驱动(硬件
- 51单片机PWM程序,占空比、周期可调整
- 单片机和adc0809数字电压表
- PIC单片机完成的电子密码锁
- 单片机、ADC0808809设计简易数字电压表
- 51单片机多功能自行车测速仪
- 单片机做的6位时分秒数字钟
- 单片机电子时钟 闹钟 日历
- 音乐代码转换软件 单片机编程时用
- 单片机课程设计 篮球计分器
- 00
- 用51单片机实现G代码翻译
- 简易的电阻电容测量电路
- 单片机与温度传感器DS18B20的程序设计
- 51单片机基于protues的几个仿真实例
- 基于52单片机的直流电压测量
- 38k单片机红外发送代码、keil
- 51单片机PCB设计图
- PC -- 单片机的串口数据传输系统设计
- 51单片机控制舵机程序
- 武汉理工大学 单片机课程设计 16*16点
- 51单片机读取温度数据存储到SD卡中并
- 【单片机】51单片机数码管显示万年历
- 浙江工业大学2005-2006学年单片机习题
- 单片机与PC机串口通讯仿真
- 毕业设计 单片机 对讲机 protues仿真
- 数据采集系统——ADC0808的应用,单片
评论
共有 条评论