资源简介
基于体渲染的OpenGL烟雾模拟程序,效率需要提高,烟雾纹理需要改进,有兴趣的朋友可以参考下!
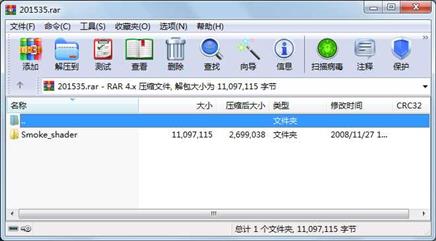
代码片段和文件信息
#include
#include “gl\glut.h“
#include
#include “maths.h“
/*-----------------------------------------------------------------
The objects motion is restricted to a rotation on a predefined axis
The function bellow does cylindrical billboarding on the Y axis i.e.
the object will be able to rotate on the Y axis only.
-----------------------------------------------------------------*/
void l3dBillboardLocalToWorld(float *cam float *worldPos) {
float modelview[16];
glGetFloatv(GL_MODELVIEW_MATRIX modelview);
// The local origin‘s position in world coordinates
worldPos[0] = cam[0] + modelview[12]*modelview[0] + modelview[13] * modelview[1] + modelview[14] * modelview[2];
worldPos[1] = cam[1] + modelview[12]*modelview[4] + modelview[13] * modelview[5] + modelview[14] * modelview[6];
worldPos[2] = cam[2] + modelview[12]*modelview[8] + modelview[13] * modelview[9] + modelview[14] * modelview[10];
}
/*-----------------------------------------------------------------
The objects motion is restricted to a rotation on a predefined axis
The function bellow does cylindrical billboarding on the Y axis i.e.
the object will be able to rotate on the Y axis only.
-----------------------------------------------------------------*/
void l3dBillboardCylindricalBegin(float *cam float *worldPos) {
float lookAt[3]={001}objToCamProj[3]upAux[3]angleCosine;
// objToCamProj is the vector in world coordinates from the local origin to the camera
// projected in the XZ plane
objToCamProj[0] = cam[0] - worldPos[0] ;
objToCamProj[1] = 0;
objToCamProj[2] = cam[2] - worldPos[2] ;
// normalize both vectors to get the cosine directly afterwards
mathsNormalize(objToCamProj);
// easy fix to determine wether the angle is negative or positive
// for positive angles upAux will be a vector pointing in the
// positive y direction otherwise upAux will point downwards
// effectively reversing the rotation.
mathsCrossProduct(upAuxlookAtobjToCamProj);
// compute the angle
angleCosine = mathsInnerProduct(lookAtobjToCamProj);
// perform the rotation. The if statement is used for stability reasons
// if the lookAt and v vectors are too close together then |aux| could
// be bigger than 1 due to lack of precision
if ((angleCosine < 0.99990) && (angleCosine > -0.9999))
glRotatef(acos(angleCosine)*180/3.14upAux[0] upAux[1] upAux[2]);
}
/*----------------------------------------------------------------
True billboarding. With the spherical version the object will
always face the camera. It requires more computational effort than
the cylindrical billboard though. The parameters camXcamY and camZ
are the target i.e. a 3D point to which the object will point.
----------------------------------------------------------------*/
void l3dBillboardSphericalBegin(float *cam float *worldPos) {
float lookAt[3]={001}objToCamProj[3]objToCam[3]upAux[3]angleCosi
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 545 2001-10-02 01:15 Smoke_shader\glutsnowman.dsw
文件 489 2001-10-17 23:18 Smoke_shader\fonts.h
文件 419 2001-11-11 04:11 Smoke_shader\l3DBillboard.h
文件 1536 2001-09-27 23:59 Smoke_shader\maths.h
文件 8661 2001-01-02 23:32 Smoke_shader\tga.cpp
文件 827 2000-12-03 00:00 Smoke_shader\tga.h
文件 262683 2000-04-02 00:45 Smoke_shader\tree.tga
文件 1864 2008-11-14 15:40 Smoke_shader\ShaderUtil.h
文件 173056 2008-12-02 16:29 Smoke_shader\glutsnowman.ncb
文件 1784 2008-12-01 16:06 Smoke_shader\glutsnowman.plg
文件 79898 1998-08-18 23:25 Smoke_shader\glut32.lib
文件 169984 1998-08-18 23:25 Smoke_shader\glut32.dll
文件 7105 2008-11-14 15:59 Smoke_shader\billboard.cpp
文件 5176 2002-05-13 15:33 Smoke_shader\spark.bmp
文件 5176 2002-05-15 16:17 Smoke_shader\bubble.bmp
文件 5176 2002-05-15 16:17 Smoke_shader\cloud.bmp
文件 2069 2008-11-14 15:35 Smoke_shader\fonts.cpp
文件 196664 2008-11-14 15:12 Smoke_shader\colorGradient.bmp
文件 192512 2006-03-04 03:19 Smoke_shader\glew32.dll
文件 281360 2006-03-04 03:19 Smoke_shader\glew32.lib
文件 7871 2008-11-14 15:35 Smoke_shader\l3dBillboard.cpp
文件 81920 2008-11-19 16:07 Smoke_shader\glutsnowman.exe
文件 8337 2008-11-14 15:40 Smoke_shader\ShaderUtil.cpp
文件 266 2008-11-14 17:19 Smoke_shader\ParticleUtils.cpp
文件 150 2008-11-14 17:19 Smoke_shader\ParticleUtils.h
文件 2310 2008-11-14 13:45 Smoke_shader\pVector.h
文件 4226 2008-11-18 10:50 Smoke_shader\glutsnowman.dsp
文件 384 2008-11-18 10:45 Smoke_shader\shader\VolumeParticle.vert
文件 169984 1998-08-18 23:25 Smoke_shader\shader\glut32.dll
文件 192512 2006-03-04 03:19 Smoke_shader\shader\glew32.dll
............此处省略49个文件信息
相关资源
- OpenGL参考手册
- Qt Creator opengl实现四元数鼠标控制轨迹
- OpenGL文档,api大全,可直接查询函数
- opengl轮廓字体源代码
- MFC读三维模型obj文件
- 利用OpenGL写毛笔字算法
- MFC中OpenGL面和体的绘制以及动画效果
- 基于OPENGL的光线跟踪源代码368758
- VC 实现三维旋转(源码)
- 自编用openGL实现3D分形树,分形山
- OpenGL球形贴图自旋程序
- OpenGL导入贴图的Texture类
- 计算机图形学(openGL)代码
- 用OpenGL开发的机械臂运动仿真程序(
- OpenGL-3D坦克模拟
- OPENGL实现世界上最小的3D游戏
- VS2012OpenGL配置所需要的全部libdllh文件
- 基于OpenGL的仿蝗虫机器人三维动态仿
- 图形学 - OpenGL实现3种三维茶壶显示源
- opengl程序-会跳舞的骷髅
- opengl实现三维网格光顺Laplacian算法
- opengl——爆炸
- OpenGL三维地形建模
- opengl游戏编程徐明亮版(含源码)
- 用OPENGL画的一个简单的直升飞机
- opengl完美天空盒
- 3D绘图程序设计:使用Direct3D 10/9和Ope
- OpenGL绘制可运动自行车源程序.zip
- OpenGL实现飘动效果
- opengl室内场景的绘制,包括碰撞检测
评论
共有 条评论