资源简介
使用TensorFlow 实现 Yolo_v1 的功能,变成语言是使用 Python3,变成环境是 Win10/Ubuntu 16.04 + TensorFlow1.4 + OpenCV 3.3,最后实现了对照片和视频的实时检测功能。具体原理参考了 Yolo_v1 的论文以及我的博客。
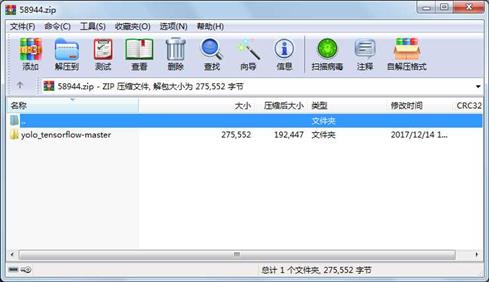
代码片段和文件信息
import tensorflow as tf
import numpy as np
import os
import cv2
import argparse
import yolo.config as cfg
from yolo.yolo_net import YOLONet
from utils.timer import Timer
class Detector(object):
def __init__(self net weight_file):
self.net = net
self.weights_file = weight_file
self.classes = cfg.CLASSES
self.num_class = len(self.classes)
self.image_size = cfg.IMAGE_SIZE
self.cell_size = cfg.CELL_SIZE
self.boxes_per_cell = cfg.BOXES_PER_CELL
self.threshold = cfg.THRESHOLD
self.iou_threshold = cfg.IOU_THRESHOLD
self.boundary1 = self.cell_size * self.cell_size * self.num_class
self.boundary2 = self.boundary1 + self.cell_size * self.cell_size * self.boxes_per_cell
self.sess = tf.Session()
self.sess.run(tf.global_variables_initializer())
print(‘Restoring weights from: ‘ + self.weights_file)
self.saver = tf.train.Saver()
self.saver.restore(self.sess self.weights_file)
def draw_result(self img result):
for i in range(len(result)):
x = int(result[i][1])
y = int(result[i][2])
w = int(result[i][3] / 2)
h = int(result[i][4] / 2)
cv2.rectangle(img (x - w y - h) (x + w y + h) (0 255 0) 2)
cv2.rectangle(img (x - w y - h - 20)
(x + w y - h) (125 125 125) -1)
cv2.putText(img result[i][0] + ‘ : %.2f‘ % result[i][5] (x - w + 5 y - h - 7)
cv2.FONT_HERSHEY_SIMPLEX 0.5 (0 0 0) 1 cv2.LINE_AA)
def detect(self img):
img_h img_w _ = img.shape
inputs = cv2.resize(img (self.image_size self.image_size))
inputs = cv2.cvtColor(inputs cv2.COLOR_BGR2RGB).astype(np.float32)
inputs = (inputs / 255.0) * 2.0 - 1.0
inputs = np.reshape(inputs (1 self.image_size self.image_size 3))
result = self.detect_from_cvmat(inputs)[0]
for i in range(len(result)):
result[i][1] *= (1.0 * img_w / self.image_size)
result[i][2] *= (1.0 * img_h / self.image_size)
result[i][3] *= (1.0 * img_w / self.image_size)
result[i][4] *= (1.0 * img_h / self.image_size)
return result
def detect_from_cvmat(self inputs):
net_output = self.sess.run(self.net.logits
feed_dict={self.net.images: inputs})
results = []
for i in range(net_output.shape[0]):
results.append(self.interpret_output(net_output[i]))
return results
def interpret_output(self output):
probs = np.zeros((self.cell_size self.cell_size
self.boxes_per_cell self.num_class))
class_probs = np.reshape(output[0:self.boundary1] (self.cell_size self.cell_size self.num_class))
scales = np.reshape(output[sel
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2017-12-14 17:09 yolo_tensorflow-master\
文件 12 2017-09-29 21:01 yolo_tensorflow-master\.gitignore
目录 0 2017-12-14 20:33 yolo_tensorflow-master\.idea\
目录 0 2017-12-11 19:06 yolo_tensorflow-master\.idea\inspectionProfiles\
文件 675 2017-12-08 12:40 yolo_tensorflow-master\.idea\inspectionProfiles\Project_Default.xm
文件 339 2017-12-08 11:02 yolo_tensorflow-master\.idea\misc.xm
文件 296 2017-12-08 11:02 yolo_tensorflow-master\.idea\modules.xm
文件 186 2017-12-11 10:24 yolo_tensorflow-master\.idea\other.xm
文件 49583 2017-12-14 20:33 yolo_tensorflow-master\.idea\workspace.xm
文件 398 2017-12-08 11:03 yolo_tensorflow-master\.idea\yolo_tensorflow-master.iml
目录 0 2017-12-08 12:29 yolo_tensorflow-master\data\
目录 0 2017-12-15 14:27 yolo_tensorflow-master\data\pascal_voc\
目录 0 2017-12-15 14:28 yolo_tensorflow-master\data\weights\
文件 306 2017-09-29 21:01 yolo_tensorflow-master\download_data.sh
文件 1067 2017-09-29 21:01 yolo_tensorflow-master\LICENSE
文件 709 2017-09-29 21:01 yolo_tensorflow-master\README.md
目录 0 2017-09-29 21:01 yolo_tensorflow-master\test\
文件 7870 2017-12-14 17:09 yolo_tensorflow-master\test.py
文件 58495 2017-09-29 21:01 yolo_tensorflow-master\test\cat.jpg
文件 113880 2017-09-29 21:01 yolo_tensorflow-master\test\person.jpg
文件 6382 2017-12-08 13:25 yolo_tensorflow-master\train.py
目录 0 2017-12-08 12:29 yolo_tensorflow-master\utils\
文件 5814 2017-12-08 12:29 yolo_tensorflow-master\utils\pascal_voc.py
文件 1130 2017-09-29 21:01 yolo_tensorflow-master\utils\timer.py
文件 0 2017-09-29 21:01 yolo_tensorflow-master\utils\__init__.py
目录 0 2017-12-08 12:29 yolo_tensorflow-master\utils\__pycache__\
文件 4824 2017-12-08 12:29 yolo_tensorflow-master\utils\__pycache__\pascal_voc.cpython-36.pyc
文件 1266 2017-12-08 11:07 yolo_tensorflow-master\utils\__pycache__\timer.cpython-36.pyc
文件 171 2017-12-08 11:07 yolo_tensorflow-master\utils\__pycache__\__init__.cpython-36.pyc
目录 0 2017-12-14 19:39 yolo_tensorflow-master\yolo\
文件 1156 2017-12-08 13:11 yolo_tensorflow-master\yolo\config.py
............此处省略6个文件信息
- 上一篇:PYQT5+图片拖拽
- 下一篇:starfm算法实现
相关资源
- 深度学习YOLOv3分类算法
- tensorflow2.0 yolo3目标检测算法
- Python-本项目基于yolo3与crnn实现中文自
- Python-基于YOLOv3的行人检测
- yolo目标检测、识别、跟踪
- yolov3-tiny检测网络
- Python-YOLOv3的PyTorch完整实现
- train_loss_acc.py
- 绘制yolov3 P-R曲线的脚本draw_pr_py3.py
- python+tensorflow的yolo实现代码
- Yolov3所需数据预处理python文件
- kmeans.py yolov3计算anchors
- yolo实现车辆识别
- qt-YOLOv3页面
-
xm
l_parse.py - yolo3物体检测源代码
- 可直接运行版本python实现yolov3调用摄
- YOLO_train.py
- 用于yolo计算mAP和PR曲线使用
- tensorflow版本的YOLO v3,在Windows系统下
- yolo_test.py
- voc to yolo标注文件格式转换器
评论
共有 条评论