资源简介
在Arduino IDE 1.0以上版本,支持采用PCF8574T芯片的国产1602显示屏。已测试。
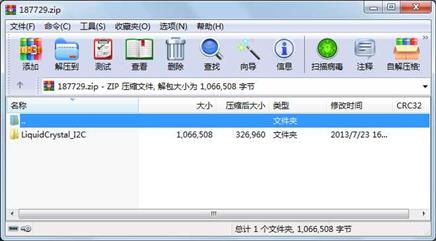
代码片段和文件信息
//YWROBOT
#include “LiquidCrystal_I2C.h“
#include
#include “Wire.h“
#include “Arduino.h“
// When the display powers up it is configured as follows:
//
// 1. Display clear
// 2. Function set:
// DL = 1; 8-bit interface data
// N = 0; 1-line display
// F = 0; 5x8 dot character font
// 3. Display on/off control:
// D = 0; Display off
// C = 0; Cursor off
// B = 0; blinking off
// 4. Entry mode set:
// I/D = 1; Increment by 1
// S = 0; No shift
//
// Note however that resetting the Arduino doesn‘t reset the LCD so we
// can‘t assume that its in that state when a sketch starts (and the
// LiquidCrystal constructor is called).
LiquidCrystal_I2C::LiquidCrystal_I2C(uint8_t lcd_Addruint8_t lcd_colsuint8_t lcd_rows)
{
_Addr = lcd_Addr;
_cols = lcd_cols;
_rows = lcd_rows;
_backlightval = LCD_NOBACKLIGHT;
}
void LiquidCrystal_I2C::init(){
init_priv();
}
void LiquidCrystal_I2C::init_priv()
{
Wire.begin();
_displayfunction = LCD_4BITMODE | LCD_1LINE | LCD_5x8DOTS;
begin(_cols _rows);
}
void LiquidCrystal_I2C::begin(uint8_t cols uint8_t lines uint8_t dotsize) {
if (lines > 1) {
_displayfunction |= LCD_2LINE;
}
_numlines = lines;
// for some 1 line displays you can select a 10 pixel high font
if ((dotsize != 0) && (lines == 1)) {
_displayfunction |= LCD_5x10DOTS;
}
// SEE PAGE 45/46 FOR INITIALIZATION SPECIFICATION!
// according to datasheet we need at least 40ms after power rises above 2.7V
// before sending commands. Arduino can turn on way befer 4.5V so we‘ll wait 50
delayMicroseconds(50000);
// Now we pull both RS and R/W low to begin commands
expanderWrite(_backlightval); // reset expanderand turn backlight off (Bit 8 =1)
delay(1000);
//put the LCD into 4 bit mode
// this is according to the hitachi HD44780 datasheet
// figure 24 pg 46
// we start in 8bit mode try to set 4 bit mode
write4bits(0x03);
delayMicroseconds(4500); // wait min 4.1ms
// second try
write4bits(0x03);
delayMicroseconds(4500); // wait min 4.1ms
// third go!
write4bits(0x03);
delayMicroseconds(150);
// finally set to 4-bit interface
write4bits(0x02);
// set # lines font size etc.
command(LCD_FUNCTIONSET | _displayfunction);
// turn the display on with no cursor or blinking default
_displaycontrol = LCD_DISPLAYON | LCD_CURSOROFF | LCD_blinkOFF;
display();
// clear it off
clear();
// Initialize to default text direction (for roman languages)
_displaymode = LCD_ENTRYLEFT | LCD_ENTRYSHIFTDECREMENT;
// set the entry mode
command(LCD_ENTRYMODESET | _displaymode);
home();
}
/********** high level commands for the user! */
void LiquidCrystal_I2C::clear(){
command(LCD_CLEARDISPLAY);// clear display set cursor position to zero
delayMicroseconds(2000); // this command takes a long time!
}
void LiquidCrystal_I2C::home()
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2013-07-23 16:50 LiquidCrystal_I2C\
目录 0 2013-07-23 15:39 LiquidCrystal_I2C\examples\
目录 0 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\
目录 0 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 93218 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 1588 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 13 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 51878 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 12090 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 9044 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 16140 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
目录 0 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 41580 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
目录 0 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 3028 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 25124 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 5588 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
目录 0 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
目录 0 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 16524 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 22756 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 8748 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 6532 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 8240 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 6416 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 4052 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 6992 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\ap
文件 1413 2013-07-23 15:39 LiquidCrystal_I2C\examples\CustomChars\CustomChars.pde
目录 0 2013-07-23 15:39 LiquidCrystal_I2C\examples\hello_2004\
目录 0 2013-07-23 15:39 LiquidCrystal_I2C\examples\hello_2004\ap
文件 93218 2013-07-23 15:39 LiquidCrystal_I2C\examples\hello_2004\ap
............此处省略55个文件信息
- 上一篇:基于FPGA病房呼叫系统
- 下一篇:s7_300_GRAPH中文编程手册
相关资源
- ADNS-3080光流传感器测试程序Arduino
- EESkill NRF24L01 无线模块用户手册
- Arduino nano 工程文件
- Arduino教程 Lesson 之--自制风扇
- 基于Arduino的智能环境监控系统设计
- 基于Arduino和Machtalk的温棚环境监测系
- arduino pca9685多舵机同时控制案例
- arduino技术内幕
- Arduino电子设计实战指南.零基础篇_超
- 物联网智能家居平台DIY:ARDUINO 物联网
- 实验1.zip arduino跑马灯led灯实验,串口
- opencat所有资料.zip
- arduino主机,stm8从机。I2C测试 。每次
- DS18B20_Serial_println.ino
- ps2手柄arduino库文件
- 基于手机蓝牙的arduino遥控小车
- arduino中的can库函数
- 密码+指纹锁资料包.rar
- 贝壳物联arduino esp8266 demo版本
- HMC5883L罗盘指南针模块库文件及中英文
- arduino 小贱钟源码及教程
- Atom-TMC2208Pilot在Arduino上运行的应用程
- 写字机制作方案
- PID-增量式PID和位置式PID算法实现和
- Building Wireless Sensor Networks Using Arduin
- vc控制Arduino,实现串口通信
- Arduino入门经典
- Arduino所有库.zip
- 基于STM32和arduino的MPU9250九轴传感器代
- GY-9960模块Keil 和Arduino驱动程序
评论
共有 条评论